I have decided to add Google Chrome browser to my browser list as test vehicle of websites I built or am going to build.
The download path is http://www.google.com/chrome/
After clicking the download button, a terms-and-conditions page appeared asking downloader to "agree and download".
The download file is called ChromeSetup.exe and it is just 551KB. Very small. It takes around a second to download the file.
ChromeSetup.exe then starts to download Google Chrome. It takes around 3 minutes to complete the download.
The installer then pops up asking me to start Google Chrome with a checkbox whether I want to make Google Chrome my default browser.
Since my Firefox is running while the installation is running, I was asked to close Firefox or skip the import of settings from Firefox.
Then the broswer pops up and the default page is Google.com. Here is the screenshot of the browser:
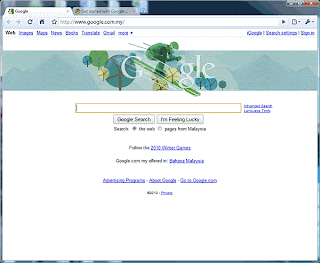
The icons appeared in my quick launch list as well as on the Desktop.
It does support my shortkey to .coms. The magic CTRL-ENTER after typing the name of the website without having to type http:// or www. or .com. Just type say "yahoo" and then press CTRL-ENTER to go to http://www.yahoo.com. A thumb up for Chrome.
For web developer, they like to "view source" and it works pretty well. But I like firefox better as it can tell you whether it is an iframe after clicking on the right button. It is still better than IE which one cannot view the source codes of an iframe.
The speed is much faster than I expected. The launching of the program is lighting fast and it is even faster than IE. I thought Safari runs the fastest but I have a new champion of speed: Chrome.
The buttons seems in the right place better than Safari which the refresh button is minute.
There is one shortcoming of Chrome that I don't like which is the inability to show the browsing history. I am unable to see the list of previous websites that I surfed. Firefox and IE are still better if this feature is compared. This could be the reason that I may not use Chrome in the future. But I will run to see the compatibility with websites I built or am going to build. This shortcoming is hurting the user-friendliness of Chrome.
In overall, the most impressive of part of Chrome is the speed. With a few more versions in the future, it should be a browser of my choice to be used on daily basis. Since the browsing history feature is lacking in this browser, I will only use it as a testing platform of websites. I hope the future releases add this feature to the speedy browser.
UPDATE: Google Chrome does have the
browsing history feature. One just need to press and hold on the forward/backward button to see the history list.
UPDATE 2 (April 4th, 2016): After five years of using Google Chrome, it is a browser I cannot live without. It is flawless when it comes to displaying website contents especially sites that run a lots of Javascripts. It is also now the top ranking browser for the visitors to this blog. It used to be the IE browser that sits in the number one spot for a long time.
Read More »