This is the touchscreen version of the post:http://webtrick101.blogspot.com/2011/12/javascript-to-move-div-around-non.htmlI believe most browser in touchscreen mobile devices will be supported such as iPhone/iPad/Android. Blackberry won't be supported as it is running browser with very low level of compatibility.Here are the codes:<html><head><script type="text/javascript">var PositionFlag = 0;var p1;var p2;var p3;var p4;var pos_x;var pos_y;var diff_x;var diff_y;var move_x;var...
Monday, December 26, 2011
Javascript to Move a DIV around (non-touchscreen version)
Ever wonder how to use javascript to move a DIV around your web page? This fascinated me because it is so cool to make your web page to have the drag-and-drop feature.The trick is to use the onmousemove event listener on the body level. Here are the codes:<html><head><script type="text/javascript">var PositionFlag = 0;var p1;var p2;var p3;var p4;var pos_x;var pos_y;var diff_x;var diff_y;var mouse_x;var mouse_y;var once = 0;function UpdateXY(){ mouse_x = window.event.clientX;...
Monday, December 5, 2011
PHP Date and Time MySQL Management Recommendation
Things are simple if everyone lives in the same time zone. Things become complicated when we want to develop a system that is "international". Time zone is a very important for internet related applications. The following shows the method I like when inserting time and date into MySQL and retrieve from it:Insert date and time to MySQL$timezone = "2"; // Get the time zone from user, usually from POST data// Normalize Date/Time to Greenwich Mean Time (GMT) Zone// Use gmdate to format to...
Tuesday, November 29, 2011
PHP to Convert Binary Data (in String Value) to Numeric Values
$string = "10101011";echo(stringbin2num($string)); // Will return 171function stringbin2num($input) { $len = strlen($input); $count = 0; $val = 0; while ($count < $len) { if (substr($input, $len-$count-1, 1)) { $val += pow(2, ($count)); } $count++; } return $val;}This function might not be needed in many situations. It's just a reference for those who want to convert string to numbers. You can also modify...
PHP to Write Binary Data (or Array Data) to a File using Pack Function
Writing the Binary Data directly using PHP's Pack() function:// Write Binary Data$bin_data = pack("C*", 0x47, 0x49, 0x46);$fp = fopen("c://test.bin", 'wb'); // replace with your filename // (please also check file permission before write)fwrite($fp, $bin_data);fclose($fp);Writing the Binary Data from an Array (if the data length is too long):// Write Binary Data$bin_data_array = array(0x47, 0x49, 0x46, .....................................);foreach ($bin_data_array...
Facebook Funny Little Trick
There are numerous posts I find it funny.It is using the Facebook wall to post something funny using the UID such as@[84786553145:0]After you paste on Facebook wall and it will appear as "Kiss Me!".It's actually showing the username of the Facebook page "Kiss Me!" and people may not be aware of this when they paste it onto their wall.But you need to add something like '+' sign to something like @+[84786553145:0] when you send the instruction to paste it to your friends wall and tell them...
Tuesday, November 22, 2011
Global Variable/Array in PHP
The global variable is declared in the function instead of in the main as in C language.<?PHP$my_global_array = array(); // Global array set up in main$my_global_variable; // Global variable set up in main...funtion a() { global $my_global_array, $my_global_variable;// declare the array and variable in main as global variable...}function b() { global $my_global_array; // only takes this global array as the global variable is not needed in this function...}?&...
Monday, November 21, 2011
CSS font used by Facebook
Now the fonts used by Facebook become the favorite for many. If you do a view source, you'll see this CSS setting:font-family:"lucida grande",tahoma,verdana,arial,sans-serif;font-size: 11px;The color tone is not entirely black and it is a bit towards gr...
PHP: Setting and Reading Cookies
<?PHPsetcookie("myCookieName", "myCookieValue"); // To read cookie, simply retrieve from the $_COOKIE['myCookieNameToRead'] ...?><HTML>..IMPORTANT: Make sure your <PHP is the first thing is your PHP file. Even a single tiny white space character prior to <PHP will also render the cookie operation usele...
PHP: $_REQUEST or $_POST or $_GET?
If you are very sure of the return string type, use $_POST['myStringKey'] for POST data and $_GET['myStringKey'] for GET data.If you are not really sure (ex. it's from a third party source such as Facebook), use $_REQUEST['myStringKey']....
PHP Weird Initialization Criteria
It is weird that before pushing data to an array, one needs to initialize the array before that.Here's what I did to avoid this weird error (pushed data will be missing):$my_array = array(); // initialize $my_array is of array typearray_push($my_array, "myTestData1");If you are used to PERL, this is wei...
The Unbelievable PHP Debug Feature: print_r()
There is a function that I personally like very much: print_r().It prints out the raw data inside a string or array. Especially when dealing nested arrays. It is a function I cannot live without.When dealing JSON data return from Facebook API/Graph, print_r() can be a very powerful debugging feature especially when the returning data structure is unknown.Here's an example of examining data returned from Facebook FQL engine:// Get your access token ($access_token) from OAuth 2.0 procedure$fql_query_url...
PHP Codes to Force a Number to Be a Negative Number
$number = ~abs($number) + 1;~ is to invert all the bits in PHP and + 1 is to make it a negative number. Remember in CS101 that binary number can be converted to represent a negative number by invert all the bits and +1? abs() is to make sure it is not a negative number and force it to be a positive number even though it is a negative number. Inverting a negative number will only make it wor...
Use JQUERY to blink Texts
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.6.4/jquery.min.js"></script><script> function startBlink(){ window.blinker = setInterval(function(){ if (window.blink) { $('.blink').css('color','black'); window.blink=false; } else { $('.blink').css('color','white'); window.blink = true; } },500); }startBlink();</script>...<span class="blink"> Your texts here! </span>This...
Sunday, November 20, 2011
The Facebook Avatar Thumb Nail URL
http://graph.facebook.com/[Facebook Profile ID]/picture
This link will be redirected to the actual jpg file in one of the servers of facebo...
PHP Code to Convert Unsigned Word (Double Byte, 16-bit String) to Signed Integer
This is useful when one tries to read a two 8-bit unsigned char from binary file and then convert to a signed integer.
The following function is good to convert from 2 character byte string such as 0A, FE, etc., to a signed integer (tested working on both 32-bit and 64-bit Windows 7 IIS environment):
function read_short($s) { // assume $s is an array of (char 1, char 2)
$temp = (($s[0]&0x00ff)<<8) + ($s[1]&0x00ff);
if ($temp & (1 << 15) ) {
$temp = ($temp...
Sunday, October 30, 2011
Setting Up A Facebook App Update (Oct 2011)
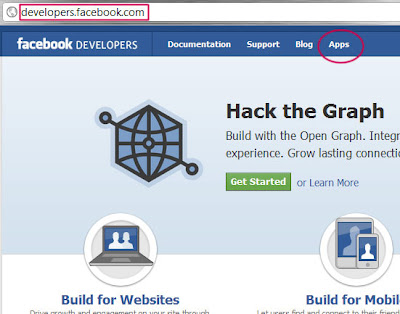
Here we go:1) Go to http://developers.facebook.com/ and click on Apps as circled below: 2) If you are here the first time, you will be asked for permission.3) You may also be asked about your phone number to verify your fb account. Do it before the next step.4) You’ll see the following wizard if you are here the first time. If you’ve set up any other apps before, you’ll be shown a different...
Friday, October 28, 2011
Facebook App Using OAuth 2.0 and Open Graph Profile

This is the path:http://www.facebook.com/apps/application.php?id=187586184649186&sk=wallNote: this is for educational purposes and the look and feel might not look professional.App Feature:- Convert your friends' contact info into csv file- You can configure particulars you like to add to the list (csv file)- Good for backing up your FB contacts- The csv file can be opened by spreadsheet software...
Thursday, October 20, 2011
Javascript to Move the HTML Body Background Image (Shake Like Wave)
Call this wave_background() function and your background will shake like you are underwater. But before that, make sure you have a background image that can mirror to x or y axis. Otherwise, the shake will look ugly.<script>var xpos = 100;var wave_timer;var tmp_string;var ran_num;var ran_dir;function wave_background() { wave_timer=setInterval("move_background()",200);}function move_background() { ran_num = Math.floor(Math.random()*5); ran_dir = Math.floor(Math.random()*6); if (ran_dir...
Monday, October 17, 2011
Add Action to Your Facebook App Before Submission for OAuth 2.0 Open Graph API
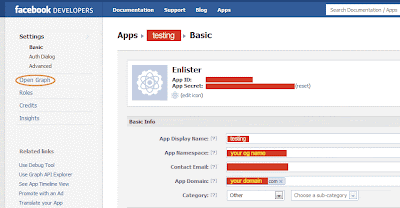
Please check out my latest update here:http://webtrick101.blogspot.com/2011/10/setting-up-facebook-app-update-oct-2011.html Some info may not be applicable any more. This latest update also covers the aggregations part in more detail.Many things have changed since the October 2011. With the Oauth 2.0, access_token is now a must. Even the hosting for the App, App's action and object are now needed...
Monday, October 10, 2011
About Installing PHP on IIS 7
All tutorials I found online are for "x86 Thread Safe" version of PHP.If you install the "Non Thread Safe" version, you won't get the php-cgi.exe in your PHP directory.So far no one points this out yet.Just in case you need this in...
Wednesday, October 5, 2011
About AJAX, JQUERY, JSON, Microsoft AJAX

Ever since facebook embraces AJAX, the popularity surged.Yahoo and Google started this game and it is good. Microsoft AJAX is having compatibility problems with browsers and JQuery took the center stage.I summarize the new trends as below so that it's easier to understand:AJAX - HTML direct connection to server without refreshing a pageJQuery - Advanced Javascript codes to manipulate CSS and AJAX...
PHP fwrite/fputs Newline Problem in IIS7
The problem I encountered is that I couldn't write a newline to the file under PC environment.Then I tried this and it worked:fwrite($fh, "My Text...\r\n");Instead of just \n, I need to have the \r\n pair under PC environment. No such issue using PERL under PC. Wei...
AJAX Using JQuery and PHP to Check Availability of User Name
Again, I compiled these codes from several sources and it's hard to give credit to all of them as some of them I only use several lines and some I only use the concept. Many I found are not fully working. That's why I want to show you the one that's working for me (I'm using PHP5 with Windows 7 via IIS7). Note: Especially the part JQUERY AJAX pass JSON data to PHP. I don't need to decode the data in PHP. If json_decode() is used, the data will be gone. I really don't know why most examples...
Free Simple Javascript Password Strength Checker
These codes came from many sources until I forgot the origins. I just combine all the good stuffs I found from the net. Sorry if I use your codes somewhere and remind me to include your credit as I forgot the origin of it.
The Javascript function:
function checkpassword(pw) {var Score = 0;
var Result = "weak";
if (pw.length>5 && pw.length<8) { Score = (Score+6); }
else if (pw.length>7 && pw.length<16) { Score = (Score+12); }
else if (pw.length>15)...
Wednesday, July 27, 2011
How to Set Facebook Landing Page to Your App?
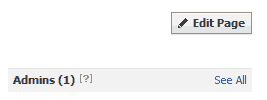
Can you do this? Yes and no.Yes, it is only applicable to those who haven't "LIKE" your page/app.No, if your fan has already "LIKE" you. They will go to the page's wall instead.You can refer to how to set up the app in my earlier post if you haven't made one yet. If you already have an app in place, you can easily go to your intended facebook page and then click on "Edit Page" in "Get Started" or...
Create a Facebook iframe App/Tab (Formerly known as Fanpage Static FMBL Tab)
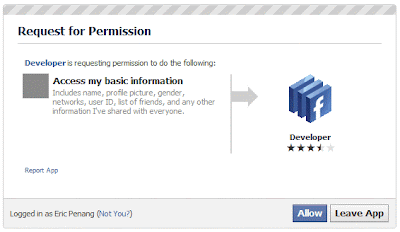
Many changes have been made recently by facebook and here's the official facebook tutorial on this subject:https://developers.facebook.com/docs/beta/opengraph/tutorial/ Many steps in the following are no longer applicable.Ever since March 11, 2011, Facebook has phased out the Static FBML support and introduced the new iframe App/Tab.As of today 27th of July, 2011, the following steps are taken to...
Wednesday, May 25, 2011
My Best Way to Place favicon.ico for All Browsers Type
Favorite Icon (favicon.ico) can help boost the professional look of your website.But somehow different browsers support it differently. Here are the codes I use for maximum compatibility:<head><link rel="icon" href="/favicon48.ico" type="image/ico"> <!-- for Mozilla based browsers --> <meta name="msapplication-task" content="name=YOUR_ICON_NAME;action-uri=./favicon32.ico"> <!-- for IE9 --> <link rel="SHORTCUT ICON" href="./favicon32.ico" type="image/x-icon">...
Sunday, May 22, 2011
How to Configure Facebook Share (fb-sahre) Thumbnail & Texts?

Update on 18/12/2014: The latest version has been updated here.
This could be something a lots of new web developers wanted to know as Facebook is gaining substantial popularity each day.If one can share its web content to Facebook, it could mean a big help to the marketing of the website.The link you need to put the Facebook link (<fb-share>) to your website or blog:<HEAD><script...
Saturday, April 2, 2011
Web Browser Usage Statistic/Market Share/Popularity
Out of my curiosity, I just check the latest stat:http://www.w3schools.com/browsers/browsers_stats.aspTo my surprise, IE is losing steam quite a lot and it is being neglected as days go by. Although Firefox is the most popular browser as of today, 2/4/11, the trend may skew towards Chrome in the near future. Good job! Google!I expected Safari to go higher due to the popularity of iPad. But to my surprise, iPad doesn't bring much to Apple in terms of the popularity of Safari used in every...
Tuesday, March 22, 2011
For IE, Don't Put CSS Style inside <TABLE>
Anything CSS style setting you put inside <TABLE> will turn into something unpredictable.Put inside <TD> instead!For Chrome, Firefox and Safari, they are OK if you put CSS style setting you put inside <TABLE&g...
Monday, March 14, 2011
Make ASP - ADODB using MS Access MDB file to display Chinese text via UTF-8
You need this:Response.CodePage = 65001somewhere in your asp code. Tada!Of course you need this in the HTML header:<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">Complete list of codepage can be found here:http://msdn.microsoft.com/en-us/library/aa752010(v=vs.85).a...
Saturday, February 12, 2011
How to get your unique Facebook URL?
Won't be able to find easily on facebook.You need to go here directly after logging to your facebook account:http://www.facebook.com/username/Very obscure and discouraged way to create a unique facebook URL of your account. Don't know the reason why but it could be to avoid abuse.Warning: Remember, you can change your username only on...
Configure your FB Likebox using CSS
Update on 31st of January 2013
This method is no longer supported. (Although the CSS names and values are still valid) Please refer to this latest method to link to the external CSS file instead:
http://webtrick101.blogspot.com/2013/01/facebook-external-css-no-longer.html
Requirement: You need to have a facebook fan page and are able to get its profile_id as explained earlier.Use this:<script src="http://connect.facebook.net/en_US/all.js#xfbml=1"></script><fb:fan profile_id="NNNNNNNNNNNNNN"...
How to build Facebook Like Box
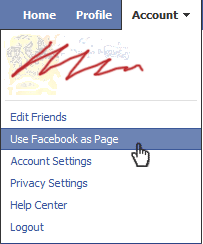
This is the method as of today as Facebook changes its features very often:0) Make sure you have a FB fan page1) Go to Account -> Use Facebook as Page (Used to be called Manage Fan Page)2) A window will pop up in the middle asking you to choose the page which you want to go to manage3) Click Edit Page on the top right on your Fan PageNote: Pay attention to the ID on your address bar (URL), something...
Sunday, January 2, 2011
IE7 & Img Onload Weird Combo (Doesn't Work and also Work)
<img src="xxx.xxx" onload="javascript:xxx();">It won't work in IE7 with Doctype set to transitional. But it works fine with or without Doctype set to transitional in IE8.It also doesn't work with iPad's Safari but works fine with PC/Mac Safari.The exclusive IE7 sniffer I like best:if (navigator.userAgent.indexOf('MSIE 7') != -1) { // Your action here!...
Subscribe to:
Posts (Atom)